Mongoose 5.7.0 was released on September 9 and is packed with new features. One major highlight is that Mongoose 5.7 supports the newly released MongoDB 4.2, which means transactions on sharded clusters. In this article, I'll cover two other major improvements: conditionally immutable properties and much faster document arrays.
Conditionally Immutable Properties
Mongoose 5.6 introduced immutable properties, which are properties that cannot change after the document is stored in the database.
const Model = mongoose.model('Test', new Schema({
createdById: {
type: Number,
immutable: true // Mark `createdById` as immutable
}
}));
const doc = await Model.create({ createdById: 1 });
// Trying to modify `createdById` is a no-op
doc.createdById = 2;
doc.createdById; // 1
await doc.save(); // Doesn't change `createdById`
// Also does nothing since `createdById` is immutable
await Model.updateOne({}, { createdById: 2 });
However, immutability in Mongoose 5.6 was all or nothing: either a property
was immutable for all instances of a model, or it wasn't. With Mongoose 5.7,
you can set the immutable
option to a function that takes the document as a parameter. For example, this means you can make a field immutable based on a user's role
:
// Only an admin can modify the number of credits they have
const User = mongoose.model('User', new Schema({
numCredits: {
type: Number,
default: 0,
immutable: doc => doc.role !== 'ADMIN'
},
role: {
type: String,
default: 'USER',
enum: ['USER', 'MODERATOR', 'ADMIN'],
immutable: true
}
}));
const user1 = await Model.create({ role: 'USER' });
user1.set({ numCredits: 25 });
user1.numCredits; // 0
const user2 = await Model.create({ role: 'ADMIN' });
user2.set({ numCredits: 25 });
user2.numCredits; // 25
Faster Document Arrays
In Mongoose 5.6.0, we overhauled Mongoose's array class to use ES6 array inheritance as opposed to mixins. In some rudimentary benchmarks this resulted in a 20% performance improvement. This change was long overdue: ES6 introduced the ability to subclass the built-in Array
class, which enables faster custom array classes. In Mongoose 5.7.0, we refactored the document array class to use ES6 array inheritance as well.
For example, consider the below script that creates a document array with 100 elements, each with 5 keys, 5000 times.
'use strict';
const mongoose = require('mongoose');
const subdocs = 100;
const reps = 5000;
const SubdocSchema = mongoose.Schema({ a: String, b: String, c: String, d: String, e: String });
const Schema = mongoose.Schema({ name: String, subdocs: { type: [SubdocSchema] } });
const Model = mongoose.model('Test', Schema);
const data = {
_id: new mongoose.Types.ObjectId(),
name: 'test',
subdocs: Array(subdocs).fill({a: 'a', b: 'b', c: 'c', d: 'd', e: 'e' })
};
const model = new Model();
for (let i = 0; i < reps; i++) {
model.init(data);
}
Mongoose 5.7 is about 35% faster than Mongoose 5.6 on the above script.
$ npm install mongoose@5.6
+ mongoose@5.6.13
added 26 packages in 3.167s
$ time node test.js
real 0m10.878s
user 0m11.444s
sys 0m0.293s
$
$ npm install mongoose@5.7
+ mongoose@5.7.0
added 1 package, removed 1 package and updated 1 package in 0.648s
$
$ time node test.js
real 0m6.940s
user 0m7.087s
sys 0m0.053s
$
Moving On
Conditionally immutable properties and faster document arrays are just 2 of the
9 new improvements and features in Mongoose 5.7.0. We also introduced MongoDB
4.2 support, middleware for query validation, and a Query#get()
function. Make sure you
upgrade to take advantage of all the new features!
Want to become your team's MongoDB expert? "Mastering Mongoose" distills 8 years of hard-earned lessons building Mongoose apps at scale into 153 pages. That means you can learn what you need to know to build production-ready full-stack apps with Node.js and MongoDB in a few days. Get your copy!
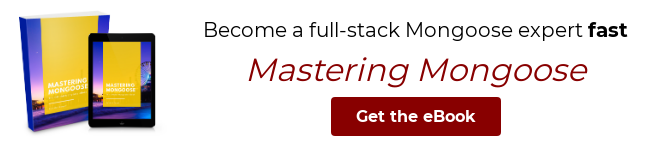